Visible to Intel only — GUID: GUID-4F0DAB12-0424-428B-86CD-99B90EA910D3
Visible to Intel only — GUID: GUID-4F0DAB12-0424-428B-86CD-99B90EA910D3
FindPeaks3x3
Finds coordinates of peaks (maximums or minimums) with absolute value exceeding threshold value.
Syntax
IppStatus ippiFindPeaks3x3_32s_C1R(const Ipp32s* pSrc, int srcStep, IppiSize roiSize, Ipp32s threshold, IppiPoint* pPeak, int maxPeakCount, int* pPeakCount, IppiNorm norm, int border, Ipp8u* pBuffer);
IppStatus ippiFindPeaks3x3_32f_C1R(const Ipp32f* pSrc, int srcStep, IppiSize roiSize, Ipp32f threshold, IppiPoint* pPeak, int maxPeakCount, int* pPeakCount, IppiNorm norm, int border, Ipp8u* pBuffer);
Include Files
ippcv.h
Domain Dependencies
Headers: ippcore.h, ippvm.h, ipps.h, ippi.h
Libraries: ippcore.lib, ippvm.lib, ipps.lib, ippi.lib
Parameters
pSrc |
Pointer to the first source image ROI. | ||||
srcStep |
Distance in bytes between starts of consecutive lines in the first source image. | ||||
roiSize |
Size of the image ROI in pixels. | ||||
threshold |
Threshold value. | ||||
pPeak |
Pointer to the coorditanes peaks [maxPeakCount]. | ||||
maxPeakCount |
Maximum number of peaks. | ||||
pPeakCount |
Pointer to the number of the detected peaks. | ||||
border |
Border value, only pixel with distance from the edge of the image greater than border are processed. | ||||
norm |
Specifies type of the norm to form the mask for extremum search:
|
||||
pBuffer |
Pointer to the working buffer. |
Description
This function operates with ROI (see Regions of Interest in Intel IPP).
This function detects local maximum and minimum pixels in the source image:
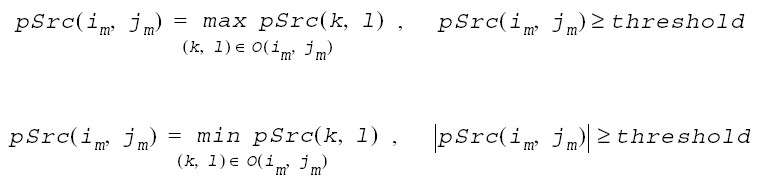
and stores their coordinates in the pPeak array pPeak[m].x = jm, pPeak[m].y = im, m = 0, ... pPeakCount[0], pPeakCount[0] ≤maxPeakCount
The neighborhood O(i, j) for the extremum search is defined by the parameter norm. The number of detected extremums is returned in pPeakCount[0]. The operation is stopped when the maxPeakCount extremums are found.
The function requires the working buffer pBuffer whose size should be computed by the function ippiFindPeaks3x3GetBufferSize beforehand.
Return Values
ippStsNoErr |
Indicates no error. Any other value indicates an error or a warning. |
ippStsNullPtrErr |
Indicates an error condition if one of the specified pointers is NULL. |
ippStsSizeErr |
Indicates an error condition if roiSize has a field with zero or negative value; or if maxPeakCount is less than or equal to 0; or if border is less than 1 or greater than one of 0.5*roiSize.width or of 0.5*roiSize.height. |
ippStsStepErr |
Indicates an error condition if srcStep is less than roiSize.width*<pixelSize>. |
ippStsNotEvenStepErr |
Indicates an error condition if srcStep is not divisible by 4. |
Example
/*******************************************************************************
* Copyright 2015 Intel Corporation.
*
*
* This software and the related documents are Intel copyrighted materials, and your use of them is governed by
* the express license under which they were provided to you ('License'). Unless the License provides otherwise,
* you may not use, modify, copy, publish, distribute, disclose or transmit this software or the related
* documents without Intel's prior written permission.
* This software and the related documents are provided as is, with no express or implied warranties, other than
* those that are expressly stated in the License.
*******************************************************************************/
// A simple example of finding coordinates of peaks
// ippiFindPeaks3x3GetBufferSize_32f_C1R
// ippiFindPeaks3x3_32f_C1R
#include <stdio.h>
#include "ipp.h"
#define WIDTH 128 /* Image width */
#define HEIGHT 128 /* Image height */
#define KERSIZE 3 /* Kernel size */
/* Next two defines are created to simplify code reading and understanding */
#define EXIT_MAIN exitLine: /* Label for Exit */
#define check_sts(st) if((st) != ippStsNoErr) goto exitLine; /* Go to Exit if Intel(R) Integrated Performance Primitives (Intel(R) IPP) function returned status different from ippStsNoErr */
/* Results of ippMalloc() are not validated because Intel(R) IPP functions perform bad arguments check and will return an appropriate status */
int main(void)
{
IppStatus status = ippStsNoErr;
Ipp32f *pSrc = NULL; /* initial image */
Ipp8u *pBuffer = NULL; /* working buffer */
IppiPoint *pPeak = NULL; /* array of peaks */
IppiSize roiSize = { WIDTH, HEIGHT }; /* image size */
int srcStep = 0; /* row step in bytes */
int bufSize = 0; /* working buffer size */
int maxPeaks=500; /* max peaks number for image */
int peakCount = 0; /* peaks number for image */
Ipp32f threshold=0.02f; /* peak threshold */
Ipp32s borderValue=40; /* Border Value */
pSrc = ippiMalloc_32f_C1(roiSize.width, roiSize.height, &srcStep);
check_sts( status = ippiFindPeaks3x3GetBufferSize_32f_C1R(roiSize.width,&bufSize) )
pBuffer = ippsMalloc_8u(bufSize);
pPeak = (IppiPoint*)ippsMalloc_8u(maxPeaks*sizeof(IppiPoint));
check_sts( status = ippiFindPeaks3x3_32f_C1R(pSrc,srcStep,roiSize,threshold,pPeak,maxPeaks,
&peakCount,ippiNormL1,borderValue,pBuffer) )
EXIT_MAIN
ippsFree(pBuffer);
ippsFree(pPeak);
ippiFree(pSrc);
printf("Exit status %d (%s)\n", (int)status, ippGetStatusString(status));
return status;
}