A.5.1. Reviewing Component Interfaces
Some interface arguments in your component can be marked as being stable. A stable interface argument is an argument that does not change while your component executes, but the argument might change between component executions. In the Component Viewer, a stable node does not have any edge connection.
Default Interface Arguments
#include "HLS/hls.h"
#include "stdio.h"
struct coordinate_t {
int x;
int y;
};
component int default_comp(int b, coordinate_t p) {
return b + p.x;
}
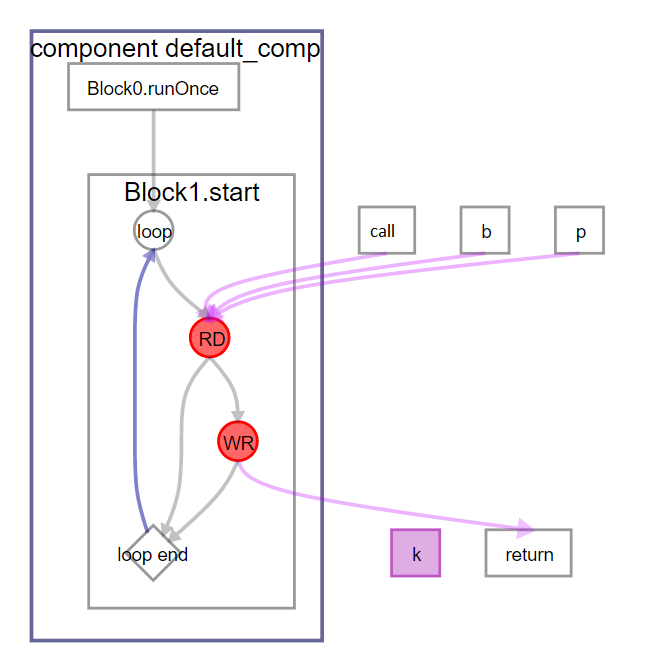
For each default interface argument node, you can view details about the node when you hover over the node:
Pointer, Pass-By-Reference, and Avalon® MM Master Interface Arguments
#include "HLS/hls.h"
#include "stdio.h"
component int master_comp(
int *pointer_d,
ihc::mm_master<int, ihc::aspace<3>, ihc::awidth<4>, ihc::dwidth<32>,ihc::latency<1>, ihc::align<4> > &master_i,
int &result
)
{
result = *pointer_d + *master_i;
return result;
}
- Stable
- Describes whether the interface argument is stable. That is, whether the hls_stable_argument attribute was applied.
- Data width
- The width of the memory-mapped data bus in bits.
- Address width
- The width of the memory-mapped address bus in bits.
- Latency
- The guaranteed latency from when the read command exits the component to when the external memory returns valid read data. The latency is measured in clock cycles.
- Maximum burst
- The maximum number of data transfers that can associate with a read or write transaction. For fixed latency interfaces, this value is set to 1.
- Alignment
- The byte alignment of the base pointer address. The Intel® HLS Compiler uses this information to determine the amount of coalescing that is possible for loads and stores to this pointer.
- Memory address space number
- The memory address space number for global memory.
- Number of banks
- The number of memory banks contained in the memory.
- Argument Name:
- The names of arguments that access the global memory.
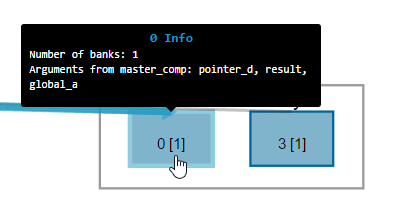
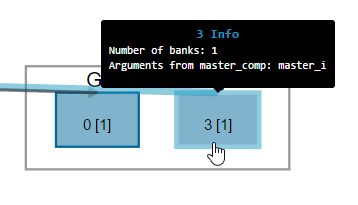
Avalon® MM Slave Register Interface Arguments
#include "HLS/hls.h"
#include "stdio.h"
component int slavereg_comp(
int hls_avalon_slave_register_argument slave_scalar_f,
int* hls_avalon_slave_register_argument slave_pointer_g
) {
return slave_scalar_f + *slave_pointer_g;
}
The resulting memory map is described in the automatically generated header file <component_name>_csr.h. This header file is available in the menu in the source code pane. Clicking on the CSR container node in the Component Viewer also opens up the header file:
#include "HLS/hls.h"
#include "stdio.h"
hls_avalon_slave_component
component int slavereg_comp(
int hls_avalon_slave_register_argument slave_scalar_f,
int* hls_avalon_slave_register_argument slave_pointer_g
) {
return slave_scalar_f + *slave_pointer_g;
}
Avalon® MM Slave Memory Interface Arguments
#include "HLS/hls.h"
#include "stdio.h"
hls_avalon_slave_component
component int slavemem_comp(
hls_avalon_slave_memory_argument(4096) int* slave_mem_h,
int index,
int hls_avalon_slave_register_argument slave_scalar_f
) {
return slave_mem_h[index] * slave_scalar_f;
}
If you look at the same Avalon® MM slave memory interface in the Component Memory Viewer report, the same <slave memory name> LD/ST node is shown to be connected to an external RW port.
Avalon® Streaming Interface Arguments
#include "HLS/hls.h"
#include "stdio.h"
component int stream_comp(
ihc::stream_in<int> &stream_in_c,
ihc::stream_out<int> &stream_out_e,
int scalar_b
) {
stream_out_e.write(scalar_b + 1);
return stream_in_c.read() + scalar_b * 2;
}
- Width
- The width of the data bus in bits.
- Depth
-
The depth of the stream in words
The word size of the stream is the size of the stream datatype.
- Bits per symbol
- Describes how the data is broken into symbols on the data bus.
- Uses Packets
- Indicates whether the interface exposes the startofpacket and endofpacket sideband signals on the stream interfaces. The signals can be access by the packet-based reads and writes.
- Uses Valid
- (stream_in) Indicates whether a valid signal is present on the stream interface. When Yes, the upstream source must provide valid data on every cycle that ready is asserted.
- Uses Reader
- (stream_in) Indicates whether a ready signal is present on the stream interface. When Yes, the downstream sink must be able to accept data on every cycle that valid is asserted.