Visible to Intel only — GUID: GUID-2FA214CF-3D76-47EC-910B-8B0E25D517C4
Visible to Intel only — GUID: GUID-2FA214CF-3D76-47EC-910B-8B0E25D517C4
AutoCorrNorm
Calculates normal, biased, and unbiased auto-correlation of a vector.
Syntax
IppStatus ippsAutoCorrNorm_32f (const Ipp32f* pSrc, int srcLen, Ipp32f* pDst, int dstLen, IppEnum algType, Ipp8u* pBuffer);
IppStatus ippsAutoCorrNorm_64f (const Ipp64f* pSrc, int srcLen, Ipp64f* pDst, int dstLen, IppEnum algType, Ipp8u* pBuffer);
IppStatus ippsAutoCorrNorm_32fc (const Ipp32fc* pSrc, int srcLen, Ipp32fc* pDst, int dstLen, IppEnum algType, Ipp8u* pBuffer);
IppStatus ippsAutoCorrNorm_64fc (const Ipp64fc* pSrc, int srcLen, Ipp64fc* pDst, int dstLen, IppEnum algType, Ipp8u* pBuffer);
Include Files
ipps.h
Parameters
pSrc |
Pointer to the source vector. |
srcLen |
Number of elements in the source vector. |
pDst |
Pointer to the destination vector. This vector stores the calculated auto-correlation of the source vector. |
dstLen |
Number of elements in the destination vector (length of auto-correlation). |
algType |
Bit-field mask for the algorithm type definition. Possible values are the results of composition of the IppAlgType and IppsNormOp values. |
pBuffer |
Pointer to the buffer for internal calculations. |
Description
Before using these functions, you need to compute the size of the work buffer using the ippsAutoCorrNormGetBufferSize function.
These functions calculate the normalized auto-correlation of the pSrc vector of srcLen length and store the results in the pDst vector of dstLen length. The result vector pDst is calculated by the following equations:
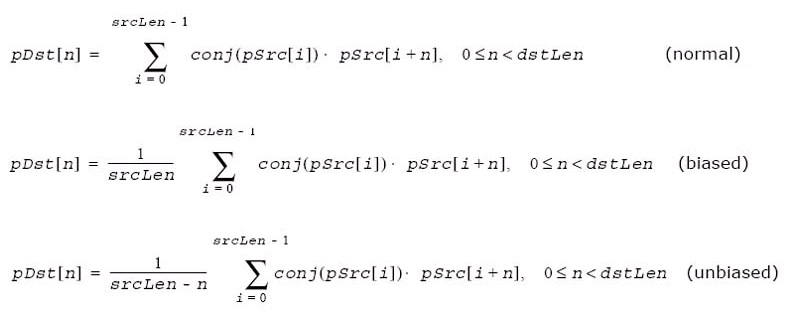
where

The auto-correlation is computed for positive lags only. Auto-correlation for a negative lag is a complex conjugate of the auto-correlation for the equivalent positive lag.
Return Values
ippStsNoErr |
Indicates no error. |
ippStsNullPtrErr |
Indicates an error when any of the specified pointers is NULL. |
ippStsSizeErr |
Indicates an error when srcLen or dstLen is less than, or equal to zero. |
ippStsAlgTypeErr |
Indicates an error when:
|
Example
The code example below demonstrates how to use the ippsAutoCorrNormGetBufferSize and ippsAutoCorrNorm functions.
IppStatus AutoCorrNormExample (void) { IppStatus status; const int srcLen = 5, dstLen = 10; Ipp32f pSrc[srcLen] = {0.2f, 3.1f, 2.0f, 1.2f, -1.1f}, pDst[dstLen]; IppEnum funCfg = (IppEnum)(ippAlgAuto|ippsNormB); int bufSize = 0; Ipp8u *pBuffer; status = ippsAutoCorrNormGetBufferSize(srcLen, dstLen, ipp32f, funCfg, &bufSize); if ( status != ippStsNoErr ) return status; pBuffer = ippsMalloc_8u( bufSize ); status = ippsAutoCorrNorm_32f(pSrc, srcLen, pDst, dstLen, funCfg, pBuffer); printf_32f("pDst", pDst, dstLen); ippsFree( pBuffer ); return status; }
The result is as follows:
pDst -> 3.3 2.0 0.6 -1.6 -0.2 0.0 0.0 0.0 0.0 0.0