Visible to Intel only — GUID: GUID-AD8D4C31-51BD-4019-AAE0-47CB8397141F
Visible to Intel only — GUID: GUID-AD8D4C31-51BD-4019-AAE0-47CB8397141F
oneapi::mkl::rng::leapfrog
Proceed state of engine using the leapfrog method.
Description
The oneapi::mkl::rng::leapfrog function generates random numbers in an engine with non-unit stride. This feature is particularly useful in distributing random numbers from the original stream across the stride buffers without generating the original random sequence with subsequent manual distribution.
One of the important applications of the leapfrog method is splitting the original sequence into non-overlapping subsequences across stride computational nodes. The function initializes the original random stream (see the following figure) to generate random numbers for the computational node idx, 0 ≤idx < stride, where stride is the largest number of computational nodes used.
Leapfrog Method
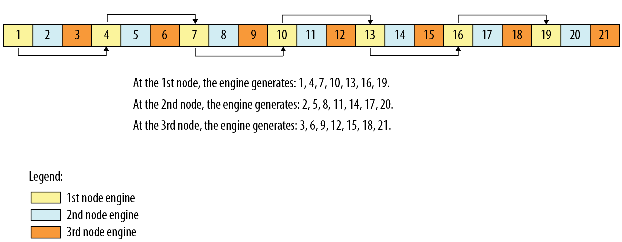
The leapfrog method is supported only for those basic generators that allow splitting elements by the leapfrog method, which is more efficient than simply generating them by a generator with subsequent manual distribution across computational nodes. See VS Notes for details.
The following code illustrates the initialization of three independent streams using the leapfrog method:
Code for Leapfrog Method
// Creating 3 identical engines mkl::rng::mcg31m1 engine_1(queue, seed); mkl::rng::mcg31m1 engine_2(engine_1); mkl::rng::mcg31m1 engine_3(engine_1); // Leapfrogging the states of engines mkl::rng::leapfrog(engine_1, 0 , 3); mkl::rng::leapfrog(engine_2, 1 , 3); mkl::rng::leapfrog(engine_3, 2 , 3); // Generating random numbers ...
API
Syntax
template<typename Engine> void leapfrog (Engine& engine, std::uint64_t idx, std::uint64_t stride)
Include Files
oneapi/mkl/rng.hpp
Input Parameters
Name |
Type |
Description |
---|---|---|
engine |
Engine |
Object of engine class, which supports leapfrog. |
idx |
std::uint64_t |
Index of the computational node. |
stride |
std::uint64_t |
Largest number of computational nodes, or stride. |