Visible to Intel only — GUID: GUID-EA3F6DA5-27E5-4DB4-BBB5-722F397BDC6A
Visible to Intel only — GUID: GUID-EA3F6DA5-27E5-4DB4-BBB5-722F397BDC6A
dot
Computes the dot product of two real vectors.
Description
The dot routines perform a dot product between two vectors. The operation is defined as:
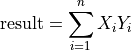
dot supports the following precisions:
T |
Tres |
---|---|
sycl::half |
sycl::half |
oneapi::mkl::bfloat16 |
oneapi::mkl::bfloat16 |
float |
float |
double |
double |
float |
double |
dot (Buffer Version)
Syntax
namespace oneapi::mkl::blas::column_major { void dot(sycl::queue &queue, std::int64_t n, sycl::buffer<T,1> &x, std::int64_t incx, sycl::buffer<T,1> &y, std::int64_t incy, sycl::buffer<Tres,1> &result) }
namespace oneapi::mkl::blas::row_major { void dot(sycl::queue &queue, std::int64_t n, sycl::buffer<T,1> &x, std::int64_t incx, sycl::buffer<T,1> &y, std::int64_t incy, sycl::buffer<Tres,1> &result) }
Input Parameters
- queue
-
The queue where the routine should be executed.
- n
-
Number of elements in vectors x and y.
- x
-
Buffer holding input vector x. Size of the buffer must be at least (1 + (n – 1)*abs(incx)). See Matrix Storage for more details.
- incx
-
Stride of vector x.
- y
-
Buffer holding input vector y. Size of the buffer must be at least (1 + (n – 1)*abs(incy)). See Matrix Storage for more details.
- incy
-
Stride of vector y.
Output Parameters
- result
-
Buffer where the result (a scalar) will be stored.
dot (USM Version)
Syntax
namespace oneapi::mkl::blas::column_major { sycl::event dot(sycl::queue &queue, std::int64_t n, const T *x, std::int64_t incx, const T *y, std::int64_t incy, Tres *result, const std::vector<sycl::event> &dependencies = {}) }
namespace oneapi::mkl::blas::row_major { sycl::event dot(sycl::queue &queue, std::int64_t n, const T *x, std::int64_t incx, const T *y, std::int64_t incy, Tres *result, const std::vector<sycl::event> &dependencies = {}) }
Input Parameters
- queue
-
The queue where the routine should be executed.
- n
-
Number of elements in vectors x and y.
- x
-
Pointer to input vector x. Size of the array holding vector x must be least (1 + (n – 1)*abs(incx)). See Matrix Storage for more details.
- incx
-
Stride of vector x.
- y
-
Pointer to input vector y. Size of the array holding vector y must be at least (1 + (n – 1)*abs(incy)). See Matrix Storage for more details.
- incy
-
Stride of vector y.
- dependencies
-
List of events to wait for before starting computation, if any. If omitted, defaults to no dependencies.
Output Parameters
- result
-
Pointer to where the result (a scalar) will be stored.
Return Values
Output event to wait on to ensure computation is complete.