Visible to Intel only — GUID: GUID-D04F50E2-FE5C-4BDE-8849-D50EC781C8A0
Visible to Intel only — GUID: GUID-D04F50E2-FE5C-4BDE-8849-D50EC781C8A0
Creating Child Windows
The FILE='USER' option in the OPEN statement opens a child window. The child window defaults to a scrollable text window, 30 rows by 80 columns. You can open up to 40 child windows.
When a file OPEN that specifies " file='user' " is issued for a program built with /libs:qwin (or /MW), special processing takes place to handle this as a graphics window. The user can then write and or read to this window by issuing READ and WRITE statements with the same unit number.
There are some api's that manipulate the window via unit number (e.g., setwsizeqq(), and others that manipulate it because focus has been set to that window. The FRTL automatically sets focus to the last window accessed. Thus, after an open, read, or write to unit number - focus is set to that window, and subsequent api calls such as setbkcolorrgb(), which sets the background color, works for this window.
Running a QuickWin application displays the frame window, but not the child window. You must call SETWINDOWCONFIG or execute an I/O statement or a graphics statement to display the child window. The window receives output by its unit number, as in:
OPEN (UNIT= 12, FILE= 'USER', TITLE= 'Product Matrix') WRITE (12, *) 'Enter matrix type: '
Child windows opened with FILE='USER' must be opened as sequential-access formatted files (the default). Other file specifications (direct-access, binary, or unformatted) result in run-time errors.
The following example creates three child windows. A frame window is automatically created. Text is written to each so the child windows are visible:
program testch use ifqwin open(11,file="user") write(11,*) "Hello 11" open(12,file="user") write(12,*) "Hello 12" open(13,file="user") write(13,*) "Hello 13" write(13,*) "Windows 11, 12, and 13 can be read and written with normal" write(13,*) "Fortran I/O statements. The size of each window on the screen" write(13,*) "can be modified by SETWSIZEQQ. The size of the virtual window" write(13,*) "(i.e., a data buffer) can be modified by SETWINDOWCONFIG." read(13,*) end
When this program is run, the output appears as follows:
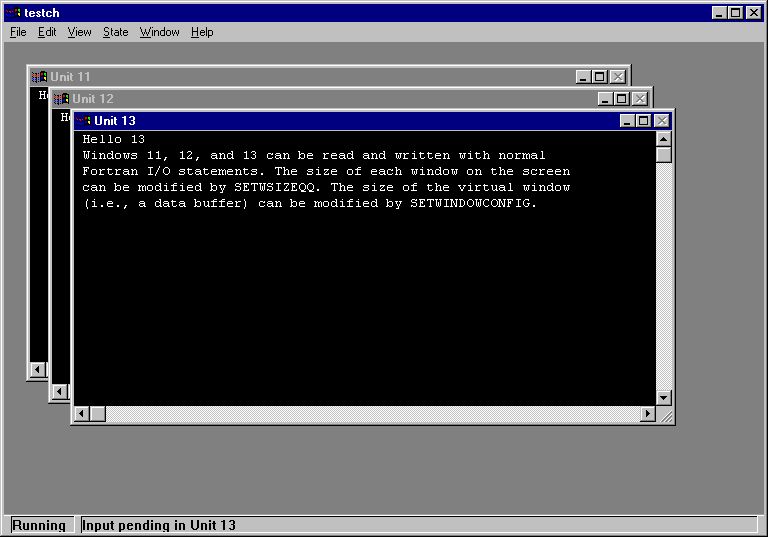
To use the default graphics window, you should use units *, 5, or 6.
In the example below, if you comment out the " open(7, …" statement, and substitute the existing unit 7's with either *, or 6 for the WRITES and 5 for the READs, then the program should behave the same. API's such as " SETWSIZEQQ(7 , …" should specify either 5 or 6, because * is not a valid integer. One issue with using a default graphics window is that you cannot change the title of it from the default title.
ifort /threads /Qsave /traceback /libs:qwin simple_example.f90
PROGRAM OneWindow USE IFQWIN USE DFMT USE DFLIB USE DFPORT type(windowconfig):: WIN_MainInput type(qwinfo) :: FrameSize, Win1Size integer(2) :: i2Status integer(4) :: i4Status,i4NumQ, i4Columns, i4LetterX, i4LetterY logical(4) :: L4Status character(1) :: c1YesNo i4LetterX=8 ! Height and width of the text font i4LetterY=16 ! ! Frame-Window as wide as screen but half the height ! i2Status=GETWSIZEQQ(QWIN$FRAMEWINDOW,QWIN$SIZEMAX,FrameSize) FrameSize.H=FrameSize.H/2 !! set window height 1/2 of max height FrameSize.W=FrameSize.W/3 !! set window width 1/3 of max width i2Status=SETWSIZEQQ(QWIN$FRAMEWINDOW,FrameSize) i4Columns=FrameSize.W/i4LetterX -1 ! number of possible columns in each window ! ! Input-window for Main part ! open(7, file='user',title="Main Input"C) Win_MainInput.title="Main Input"C !! in addition to OPEN, title can also be specified by setwindowconfig() Win_MainInput.numtextrows=INT((FrameSize.H-40)/i4LetterY) Win_MainInput.numtextcols=i4Columns Win_MainInput.fontsize=65536*i4LetterX+i4LetterY L4Status=setwindowconfig(Win_MainInput) i4Status=setbkcolorrgb(#FFFFFF) !! white background i4Status=settextcolorrgb(#000000) !! black text call clearscreen($GCLEARSCREEN) Win1Size.X=0 Win1Size.Y=0 Win1Size.H=INT((FrameSize.H-40)/i4LetterY) Win1Size.W=i4Columns Win1Size.type=QWIN$SET i4Status=setwsizeqq(7,Win1Size) write(7,'("This is the Main Input window.",/)') 10 write(7,'("Type Y or y to Terminate this program: ",\)') read(7,'(Q,A)') i4NumQ, c1YesNo if (i4NumQ /= 1) goto 10 if (c1YesNo == 'Y' .or. c1YesNo == 'y') goto 20 goto 10 20 continue END PROGRAM OneWindow