Visible to Intel only — GUID: GUID-36473950-3294-4C8A-92A5-E6F9BD64373F
Visible to Intel only — GUID: GUID-36473950-3294-4C8A-92A5-E6F9BD64373F
C Interface Conventions
The C interfaces are implemented for most of the oneMKL LAPACK driver and computational routines.
The arguments of the C interfaces for the oneMKL LAPACK functions comply with the following rules:
Scalar input arguments are passed by value.
Array arguments are passed by reference.
Array input arguments are declared with the const modifier.
Function arguments are passed by pointer.
An integer return value replaces the info output parameter. The return value equal to 0 means the function operation is completed successfully. See the section Error Codes below.
Most of the LAPACK C interfaces have an additional parameter matrix_layout of the int type as their first argument. This parameter specifies whether the two-dimensional arrays are row-major (LAPACK_ROW_MAJOR) or column-major (LAPACK_COL_MAJOR).
The LAPACK C interface omits workspace parameters because workspace is allocated at run time and released upon completion of the function operation.
For the ?gesvd function, work arrays contain valuable information on exit. The interface contains an additional argument superb.
Function Types
The function types are used in non-symmetric eigenproblem functions only.
typedef lapack_logical (*LAPACK_S_SELECT2) (const float*, const float*); typedef lapack_logical (*LAPACK_S_SELECT3) (const float*, const float*, const float*); typedef lapack_logical (*LAPACK_D_SELECT2) (const double*, const double*); typedef lapack_logical (*LAPACK_D_SELECT3) (const double*, const double*, const double*); typedef lapack_logical (*LAPACK_C_SELECT1) (const lapack_complex_float*); typedef lapack_logical (*LAPACK_C_SELECT2) (const lapack_complex_float*, const lapack_complex_float*); typedef lapack_logical (*LAPACK_Z_SELECT1) (const lapack_complex_double*); typedef lapack_logical (*LAPACK_Z_SELECT2) (const lapack_complex_double*, const lapack_complex_double*);
Mapping FORTRAN Data Types against C Data Types
FORTRAN Data Types | C Data Types |
---|---|
INTEGER | lapack_int |
LOGICAL | lapack_logical |
REAL | float |
DOUBLE PRECISION | double |
COMPLEX | lapack_complex_float |
COMPLEX*16 | lapack_complex_double |
CHARACTER | char |
C Types
#ifndef lapack_int #define lapack_int MKL_INT #endif #ifndef lapack_logical #define lapack_logical lapack_int #endif
Complex Types
For single precision:
#ifndef lapack_complex_float #define lapack_complex_float MKL_Complex8 #endif
For double precision:
#ifndef lapack_complex_double #define lapack_complex_double MKL_Complex16 #endif
Matrix Layout
Most of the LAPACK C interfaces have an additional parameter matrix_layout of type int as their first argument. This parameter specifies whether the two-dimensional arrays are row-major LAPACK_ROW_MAJOR or column-major LAPACK_COL_MAJOR.
In general the leading dimension lda is equal to the number of elements in the major dimension. It is also equal to the distance in elements between two neighboring elements in a line in the minor dimension. If there are no extra elements in a matrix with m rows and n columns, then
- For row-major ordering: the number of elements in a row is n, and row i is stored in memory right after row i-1. Therefore lda is n.
- For column-major ordering: the number of elements in a column is m, and column i is stored in memory right after column i-1. Therefore lda is m.
To refer to a submatrix with dimensions k by l, use the number of elements in the major dimension of the whole matrix (as above) as the leading dimension and k and l in the subroutine's input parameters to describe the size of the submatrix.
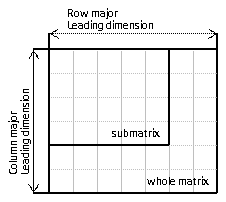
Matrix Ordering
#define LAPACK_ROW_MAJOR 101 #define LAPACK_COL_MAJOR 102
Error Codes
#define LAPACK_WORK_MEMORY_ERROR -1010 /* Failed to allocate memory for a working array */ #define LAPACK_TRANSPOSE_MEMORY_ERROR -1011 /* Failed to allocate memory for transposed matrix */
If the return value is -i, the -i-th parameter has an invalid value.
Function Prototypes
Some oneMKL functions differ in data types they support and vary in the parameters they take.
Each function type has a unique prototype defined. Use this prototype when you call the function from your application program. In most cases, oneMKL supports four distinct floating-point precisions. Each corresponding prototype looks similar, usually differing only in the data type. To avoid listing all the prototypes in every supported precision, a generic prototype template is provided. For example,
lapack_int LAPACKE_