Intel® Integrated Performance Primitives (Intel® IPP) Developer Guide and Reference
A newer version of this document is available. Customers should click here to go to the newest version.
Visible to Intel only — GUID: GUID-40E2F16A-B48E-4D10-995F-4323AF38F11A
Visible to Intel only — GUID: GUID-40E2F16A-B48E-4D10-995F-4323AF38F11A
CopyReplicateBorder
Copies pixels values between two images and adds the replicated border pixels.
Syntax
Case 1: Not-in-place operation
IppStatus ippiCopyReplicateBorder_<mod>(const Ipp<datatype>* pSrc, int srcStep, IppiSize srcRoiSize, Ipp<datatype>* pDst, int dstStep, IppiSize dstRoiSize, int topBorderHeight, int leftBorderWidth);
Supported values for mod:
8u_C1R | 16u_C1R | 16s_C1R | 32s_C1R | 32f_C1R |
8u_C3R | 16u_C3R | 16s_C3R | 32s_C3R | 32f_C3R |
8u_C4R | 16u_C4R | 16s_C4R | 32s_C4R | 32f_C4R |
8u_AC4R | 16u_AC4R | 16s_AC4R | 32s_AC4R | 32f_AC4R |
Case 2: In-place operation
IppStatus ippiCopyReplicateBorder_<mod>(const Ipp<datatype>* pSrc, int srcDstStep, IppiSize srcRoiSize, IppiSize dstRoiSize, int topBorderHeight, int leftBorderWidth);
Supported values for mod:
8u_C1IR | 16u_C1IR | 16s_C1IR | 32s_C1IR | 32f_C1IR |
8u_C3IR | 16u_C3IR | 16s_C3IR | 32s_C3IR | 32f_C3IR |
8u_C4IR | 16u_C4IR | 16s_C4IR | 32s_C4IR | 32f_C4IR |
8u_AC4IR | 16u_AC4IR | 16s_AC4IR | 32s_AC4IR | 32f_AC4IR |
Case 3: Not-in-place operation for platform-aware functions
IppStatus ippiCopyReplicateBorder_<mod>(const Ipp<datatype>* pSrc, IppSizeL srcStep, IppiSizeL srcRoiSize, Ipp<datatype>* pDst, IppSizeL dstStep, IppiSizeL dstRoiSize, IppSizeL topBorderHeight, IppSizeL leftBorderWidth);
Supported values for mod:
8u_C1R_L | 16u_C1R_L | 16s_C1R_L | 32s_C1R_L | 32f_C1R_L |
8u_C3R_L | 16u_C3R_L | 16s_C3R_L | 32s_C3R_L | 32f_C3R_L |
8u_C4R_L | 16u_C4R_L | 16s_C4R_L | 32s_C4R_L | 32f_C4R_L |
8u_AC4R_L | 16u_AC4R_L | 16s_AC4R_L | 32s_AC4R_L | 32f_AC4R_L |
Case 4: In-place operation for platform-aware functions
IppStatus ippiCopyReplicateBorder_<mod>(const Ipp<datatype>* pSrc, IppSizeL srcDstStep, IppiSizeL srcRoiSize, IppiSizeL dstRoiSize, IppSizeL topBorderHeight, IppSizeL leftBorderWidth);
Supported values for mod:
8u_C1IR_L | 16u_C1IR_L | 16s_C1IR_L | 32s_C1IR_L | 32f_C1IR_L |
8u_C3IR_L | 16u_C3IR_L | 16s_C3IR_L | 32s_C3IR_L | 32f_C3IR_L |
8u_C4IR_L | 16u_C4IR_L | 16s_C4IR_L | 32s_C4IR_L | 32f_C4IR_L |
8u_AC4IR_L | 16u_AC4IR_L | 16s_AC4IR_L | 32s_AC4IR_L | 32f_AC4IR_L |
Include Files
ippi.h
Flavors with the _L suffix: ippi_l.h
Domain Dependencies
Headers: ippcore.h, ippvm.h, ipps.h
Libraries: ippcore.lib, ippvm.lib, ipps.lib
Parameters
pSrc |
Pointer to the source image ROI. |
srcStep |
Distance in bytes between starts of consecutive lines in the source image. |
pDst |
Pointer to the destination image. |
dstStep |
Distance in bytes between starts of consecutive lines in destination image. |
srcDstStep |
Distance in bytes between starts of consecutive lines in the source and the destination image. |
srcRoiSize |
Size of the source ROI in pixels. |
dstRoiSize |
Size of the destination ROI in pixels. |
topBorderHeight |
Height of the top border in pixels. |
leftBorderWidth |
Width of the left border in pixels. |
Description
This function operates with ROI (see Regions of Interest in Intel IPP).
This function copies the source image pSrc to the destination image pDst and fills pixels ("border") outside the copied area in the destination image with the values of the source image pixels according to the scheme illustrated in Figure Creating a Replicated Border. Squares marked in red correspond to pixels copied from the source image, that is, the source image ROI.
Note that the in-place function flavor actually adds border pixels to the source image ROI, thus a destination image ROI is larger than the initial image.
The parameters topBorderHeight and leftBorderWidth specify the position of the first pixel of the source ROI in the destination image ROI.
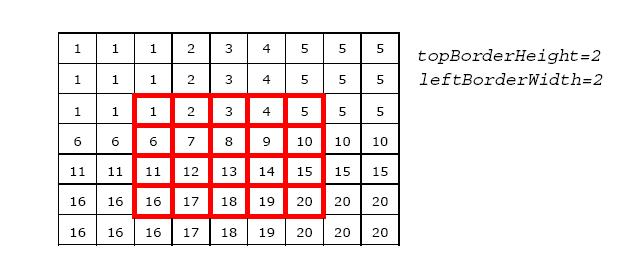
The height (width) of the destination ROI cannot be less than the sum of the height (width) of source ROI and the topBorderHeight (leftBorderWidth) parameter.
Return Values
ippStsNoErr |
Indicates no error. Any other value indicates an error or a warning. |
ippStsNullPtrErr |
Indicates an error when any of the specified pointers is NULL. |
ippStsSizeErr |
Indicates an error condition if srcRoiSize or dstRoiSize has a field with a zero or negative value, or topBorderHeight or leftBorderWidth is less than zero, or dstRoiSize.width < srcRoiSize.width + leftBorderWidth, or dstRoiSize.height < srcRoiSize.height + topBorderHeight. |
ippStsStepErr |
Indicates an error condition if srcStep or dstStep has a zero or negative value. |
Example
The code example below shows how to use the ippiCopyReplicateBorder_8u_C1R function.
Ipp8u src[8*4] = {5, 4, 3, 4, 5, 8, 8, 8, 3, 2, 1, 2, 3, 8, 8, 8, 3, 2, 1, 2, 3, 8, 8, 8, 5, 4, 3, 4, 5, 8, 8, 8}; Ipp8u dst[9*8]; IppiSize srcRoi = { 5, 4 }; IppiSize dstRoi = { 9, 8 }; int topborderHeight = 2; int leftborderWidth = 2; ippiCopyReplicateBorder_8u_C1R(src, 8, srcRoi, dst, 9, dstRoi, topBorderHeight, leftBorderWidth);
Results
source image:
5 4 3 4 5 8 8 8
3 2 1 2 3 8 8 8
3 2 1 2 3 8 8 8
5 4 3 4 5 8 8 8
destination image:
5 5 5 4 3 4 5 5 5
5 5 5 4 3 4 5 5 5
5 5 5 4 3 4 5 5 5
3 3 3 2 1 2 3 3 3
3 3 3 2 1 2 3 3 3
5 5 5 4 3 4 5 5 5
5 5 5 4 3 4 5 5 5
5 5 5 4 3 4 5 5 5